If you find yourself wanting to spawn a Blueprint class within C++, here’s how.
Read More →Code Snippets
Visual Studio Extensions: How to get colors from the VS theme
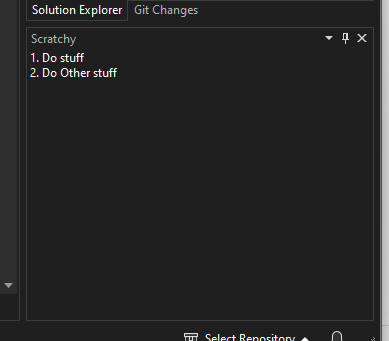
TL;DR:
// Note that I've only tried this in VS 2022.
// Browse the EnvironmentColors class to see all the available colors.
var themeColor = VSColorTheme.GetThemedColor(EnvironmentColors.ToolWindowBackgroundColorKey);
// If you want to use this in a WPF brush, convert it like this.
var wpfCompatibleColor = Color.FromRgb(themeColor.R, themeColor.G, themeColor.B);
Read on for full details.
Read More →console.log() with jsFiddle
jsFiddle is a great tool for quick JavaScript prototyping. While it’s possible to use your browser’s console log to preview bits and pieces of output, I was looking for a cleaner way to do this, using just the jsFiddle panes. I’ve come up with a simple way to add a logging facility to the Result pane if you are using jQuery.
JavaScript snippet: Remove base URL from link
I needed this function this morning so I thought I’d share it in case someone else does too.
function RemoveBaseUrl(url) {
/*
* Replace base URL in given string, if it exists, and return the result.
*
* e.g. "http://localhost:8000/api/v1/blah/" becomes "/api/v1/blah/"
* "/api/v1/blah/" stays "/api/v1/blah/"
*/
var baseUrlPattern = /^https?:\/\/[a-z\:0-9.]+/;
var result = "";
var match = baseUrlPattern.exec(url);
if (match != null) {
result = match[0];
}
if (result.length > 0) {
url = url.replace(result, "");
}
return url;
}